Methods thông dụng của collection trong laravel
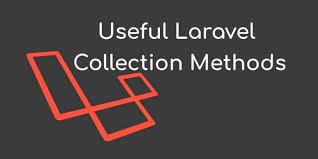
Trong laravel sử dụng Collection giúp lập trình viên có thể xử lý nhanh chóng dữ liệu trả về sau khi truy vấn Eloquent. Để sử dụng nó, bạn cần import class Illuminate\Support\Collection
và dùng collect
helper để khởi tạo mới một đối tượng.
Nội dung
1. All() : Lấy ra tất các giá trị trong 1 collection.
Ví dụ:
$collectionAll = collect([
[
'user_id' => '1',
'title' => 'Helpers in Laravel',
'content' => 'Create custom helpers in Laravel',
'category' => 'php'
],
[
'user_id' => '2',
'title' => 'Testing in Laravel',
'content' => 'Testing File Uploads in Laravel',
'category' => 'php'
],
[
'user_id' => '3',
'title' => 'Telegram Bot',
'content' => 'Crypto Telegram Bot in Laravel',
'category' => 'php'
],
]);
$checkAll = collect($collectionAll)->all();
Kết quả:
2.Filter() : Lấy ra các giá trị thỏa mãn 1 điều kiện nhất định.
Ví dụ :
$filter = $collectionAll->filter(function($item) {
if ($item['user_id'] == 2) {
return true;
}
});
$checkFilter = $filter->all();
Kết quả :
3. Duplicate() : Lấy ra các phần tử trùng và nếu có 2 phần tử cùng giá trị thì lấy phần tử cuối cùng trường hợp có tham số đầu vào.
Ví dụ :
$collDuplicateTwo = collect([
['model' => 'A8', 'Brand' => 'Audi'],
['model' => 'W150', 'Brand' => 'Mercedes'],
['model' => 'W150', 'Brand' => 'Maruti'],
]);
$checkDuplicateTwo = $collDuplicateTwo->duplicates('model')->toArray();
Kết quả :
4. Only() : Lọc các giá trị trong collection theo keys
Ví dụ :
$collectionOnly = collect(['name' => 'hoanpham', 'gender' => 'Male', 'age' => 24, 'Nationality' => 'VN']);
$checkOnly = $collectionOnly->only(['name','gender'])->toArray();
Kết quả :
5. Map() : lọc qua các phần tử của collection và tại đây có thể thay đổi giá trị trả về
Ví dụ :
$collectionMap = collect([1, 2, 3, 4, 5]);
$multiplied = $collectionMap->map(function ($item, $key) {
return $item * 2;
});
Kết quả :
6. Reject() : Loại bỏ các giá trị trong collection nếu thoả mãn điều kiện cho trước
Ví dụ :
$collectionReject = collect([1, 2, 3, 4]);
$filtered = $collectionReject->reject(function ($item) {
return $item > 2;
});
Kết quả :
7. Each() : Duyệt qua các phần tử của collection đồng thời bạn có thể thoát khỏi vòng lặp nếu xét và thỏa mãn điều kiện
Ví dụ :
$collectionEach = collect([ 'London', 'Paris', 'New York', 'Toranto', 'Tokyo']);
$checkEach = [];
$collectionEach->each(function ($item) use (&$checkEach) {
if ($item == 'Paris') {
return false;
}
array_push($checkEach, $item);
});
Kết quả :
8. GroupBy() : Gom nhóm các giá trị của collection cùng 1 key
Ví dụ:
$collectionGroupBy = collect([
['name' => 'Hoan1' , 'age' => 24, 'country' => 'VN'],
['name' => 'Hoan1' , 'age' => 24, 'country' => 'VN1'],
['name' => 'Hoan3' , 'age' => 26, 'country' => 'VN'],
['name' => 'Hoan4' , 'age' => 27, 'country' => 'VN']
]);
$checkGroupByTwo = $collectionGroupBy->groupBy(function ($item , $key) {
return $item['name'] . $item['country'] . $key;
});
Kết quả:
9. Join() : Nối các giá trị trong 1 item thành 1 string
Ví dụ :
$collectionJoin = collect(['a', 'b', 'c']);
$checkJoin = $collectionJoin->join(', ', ', and ');
Kết quả :
10. KeyBy() : Lọc ra các giá trị trong collection theo key và nếu các item có cùng key thì giá trị item cuối sẽ được lấy
Ví dụ:
$collectionKeyBy = collect([
['product_id' => 'prod-100', 'name' => 'Desk'],
['product_id' => 'prod-200', 'name' => 'Chair'],
['product_id' => 'prod-200', 'name' => 'Chair2'],
]);
$keyed = $collectionKeyBy->keyBy('product_id')->toArray();
Kết quả:
11. Merge() : tạo ra 1 collection mới từ việc gom nhóm các phần tử trong các collection cũ
Ví dụ :
$collectionMerged = collect(['HoanOne', 'HoanTwo']);
$merged = $collectionMerged->merge(['HoanThree', 'HoanFour']);
Kết quả :
12. Pluck() : lọc ra các giá trị theo key
Ví dụ :
$collectionPluck = collect([
['product_id' => 'prod-100', 'name' => 'Desk'],
['product_id' => 'prod-200', 'name' => 'Chair'],
['product_id' => 'prod-200', 'name' => 'Chair2'],
]);
$plucked = $collectionPluck->pluck('name');
Kết quả :
13. Reduce() : tái tạo các giá trị trong collection thành 1 giá trị đơn
Ví dụ :
$collectionReduce = collect([
'hoanOne' => 1000,
'hoanTwo' => 1000,
'hoanThree' => 1000,
]);
$ratio = [
'hoanOne' => 1,
'hoanTwo' => 2,
'hoanThree' => 3,
];
$checkReduce = $collectionReduce->reduce(function ($carry, $value, $key) use ($ratio) {
return $carry + ($value * $ratio[$key]);
});
Kết quả :
Tài liệu tham khảo
https://codezen.io/most-useful-laravel-collection-methods/
https://laravel.com/docs/8.x/collections#method-reduce
https://www.pakainfo.com/laravel-6-collection-methods-with-examples/