Routing trong Angular
Như bạn đã biết Angular là một Javascript Framework để xây dựng các Single Page Application (SPA) bằng JavaScript, HTML và TypeScript. Giả sử bạn muốn thiết kế các trang như Home page, contact us, help, policy. Làm thế nào để bạn đạt được điều này trong Angular?
Để tạo được các liên kết trong Angular, bạn có thể sử dụng Routing. Routing trong Angular về cơ bản có nghĩa là điều hướng giữa các trang. Ở đây, các trang mà chúng ta đang đề cập đến sẽ ở dạng các Component. Bây giờ chúng ta sẽ tạo một component và xem cách sử dụng routing với nó.
Khi tạo project bằng lệnh ng new ten_project
, hệ thống hỏi chúng ta có muốn tạo routing hay không? Nếu nhập yes là có. Có nghĩa là Routing Module được thêm vào file app.module.ts như sau:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | import { BrowserModule } from '@angular/platform-browser' ; import { NgModule } from '@angular/core' ; import { AppRoutingModule } from './app-routing.module' ; import { AppComponent } from './app.component' ; import { NewCmpComponent } from './new-cmp/new-cmp.component' ; import { AddTextDirective } from './add-text.directive' ; import { SqrtPipe, SquarePipe } from './app.sqrt' ; @NgModule({ declarations: [ AppComponent, NewCmpComponent, AddTextDirective, SqrtPipe, SquarePipe ], imports: [ BrowserModule, AppRoutingModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { } |
AppRoutingModule được thêm vào như hình trên và được đưa vào mảng imports.
Chi tiết tệp của app-routing.module được cung cấp bên dưới:
1 2 3 4 5 6 7 8 9 10 | import { NgModule } from '@angular/core' ; import { Routes, RouterModule } from '@angular/router' ; const routes: Routes = []; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { } |
Lưu ý file này được tạo theo mặc định với tùy chọn thêm routing vào trong quá trình thiết lập dự án. Nếu không được thêm, các tệp trên phải được thêm theo cách thủ công.
Có một const route được định nghĩa là kiểu Routes. Nó là một mảng chứa tất cả các path mà chúng ta cần trong dự án của mình.
const routes được cấp cho RouterModule như được hiển thị trong @NgModule. Để hiển thị chi tiết định tuyến cho người dùng, chúng ta cần thêm chỉ thị <router-outlet> vào view (html, template) được hiển thị.
Ví dụ <router-outlet> được thêm vào app.component.html như sau:
1 2 | <h1>Angular 7 Routing Demo</h1> <router-outlet></router-outlet> |
Ví dụ sử dụng routing trong Angular
Bây giờ chúng ta sẽ tạo 2 component được gọi là Home và ContactUs và điều hướng giữa chúng bằng cách sử dụng routing.
Tạo Home component
Thực thi lệnh sau để tạo home component:
ng g component home
Tạo ContactUs component
Thực thi lệnh sau để tạo ContactUs component:
ng g component contactus
Chúng ta đã hoàn tất việc tạo các component về home và contactus. Dưới đây là chi tiết về các thành phần trong app.module.ts
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | import { BrowserModule } from '@angular/platform-browser' ; import { NgModule } from '@angular/core' ; import { AppRoutingModule } from './app-routing.module' ; import { AppComponent } from './app.component' ; import { NewCmpComponent } from './new-cmp/new-cmp.component' ; import { AddTextDirective } from './add-text.directive' ; import { SqrtPipe, SquarePipe } from './app.sqrt' ; import { HomeComponent } from './home/home.component' ; import { ContactusComponent } from './contactus/contactus.component' ; @NgModule({ declarations: [ AppComponent, NewCmpComponent, AddTextDirective, SqrtPipe, SquarePipe, HomeComponent, ContactusComponent ], imports: [ BrowserModule, AppRoutingModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { } |
Import RoutingComponent vào app.module.ts như sau:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | import { BrowserModule } from '@angular/platform-browser' ; import { NgModule } from '@angular/core' ; import { AppRoutingModule, RoutingComponent } from './app-routing.module' ; import { AppComponent } from './app.component' ; import { NewCmpComponent } from './new-cmp/new-cmp.component' ; import { AddTextDirective } from './add-text.directive' ; import { SqrtPipe, SquarePipe } from './app.sqrt' ; import { HomeComponent } from './home/home.component' ; import { ContactusComponent } from './contactus/contactus.component' ; @NgModule({ declarations: [ AppComponent, NewCmpComponent, AddTextDirective, SqrtPipe, SquarePipe, HomeComponent, ContactusComponent, RoutingComponent ], imports: [ BrowserModule, AppRoutingModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { } |
Bây giờ chúng ta hãy thêm chi tiết các path trong app-routing.module.ts như sau:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | import { NgModule } from '@angular/core' ; import { Routes, RouterModule } from '@angular/router' ; import { HomeComponent } from './home/home.component' ; import { ContactusComponent } from './contactus/contactus.component' ; const routes: Routes = [ {path: "home" , component:HomeComponent}, {path: "contactus" , component:ContactusComponent} ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { } |
Mảng các routes gồm có các path và component.
Lưu ý: các component chúng ta cần để định tuyến được import trong app.module.ts và cả trong app-routing.module.ts. Bạn có thể chỉ cần import chúng 1 nơi, tức là trong app-routing.module.ts.
Vì vậy, chúng tôi sẽ tạo một mảng component được sử dụng để định tuyến và sẽ export mảng trong app-routing.module.ts và import lại nó trong app.module.ts. Vì vậy, chúng ta có tất cả các component được sử dụng để định tuyến trong app-routing.module.ts.
app-routing.module.ts như sau:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | import { NgModule } from '@angular/core' ; import { Routes, RouterModule } from '@angular/router' ; import { HomeComponent } from './home/home.component' ; import { ContactusComponent } from './contactus/contactus.component' ; const routes: Routes = [ {path: "home" , component:HomeComponent}, {path: "contactus" , component:ContactusComponent} ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { } export const RoutingComponent = [HomeComponent,ContactusComponent]; |
Chúng ta đã hoàn tất việc định nghĩa các routing. Bây giờ, chúng ta cần hiển thị giống nhau cho người dùng, vì vậy hãy thêm hai button, home và contact us trong app.component.html và khi click vào button tương ứng, nó sẽ hiển thị template(.html) component tương ứng bên trong chỉ thị <router-outlet> mà chúng ta đã thêm vào add.component.html.
Tạo button bên trong app.component.html và cung cấp đường dẫn đến các routing đã tạo.
app.component.html
1 2 3 4 5 6 | <h1>Angular 7 Routing Demo</h1> <nav> <a routerLink = "/home" >Home</a> <a routerLink = "/contactus" >Contact Us </a> </nav> <router-outlet></router-outlet> |
Thêm css sau vào app.component.css
1 2 3 4 5 6 7 8 9 10 11 | a:link, a:visited { background-color: #848686; color: white; padding: 10px 25px; text-align: center; text-decoration: none; display: inline-block; } a:hover, a:active { background-color: #BD9696; } |
Kết quả:
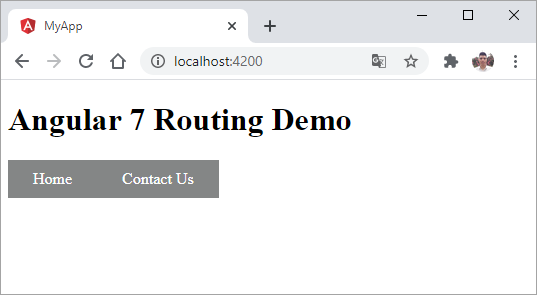
Click Home:
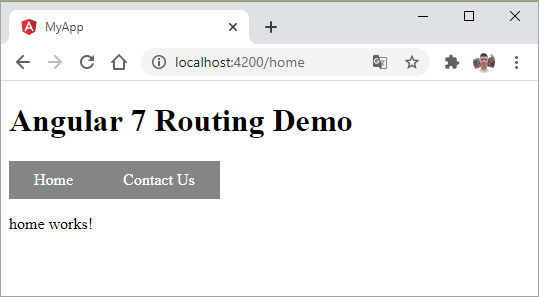
Click Contact Us:
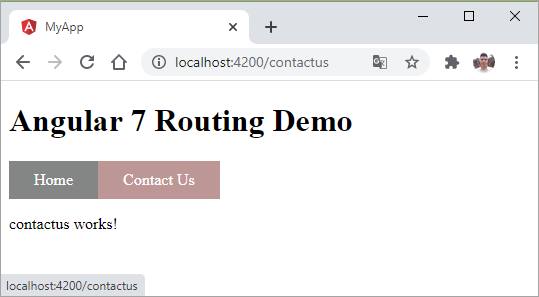