Những thay đổi trong PHP 8
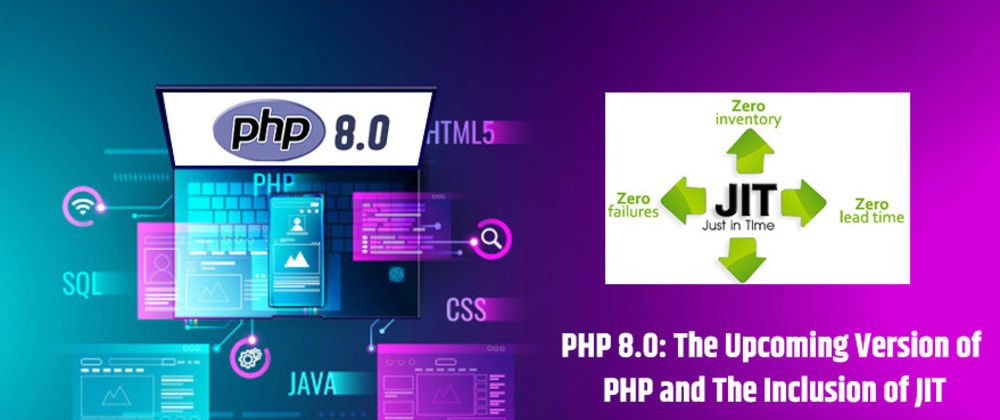
PHP 8 dự kiến chính thức ra mắt vào 26/11/2020 với nhiều thay đổi khi có thêm nhiều chức năng mới, cũng như có những cải thiện về hiệu năng. Cùng điểm qua một số thay đổi trong PHP 8
1. Union types
- Union types là tập hợp bao gồm 1 hoặc nhiều types(ngoại trừ Void) => data của bạn có kiểu dữ liệu nằm trong tập hợp đấy
public function foo(Foo|Bar $input): int|float;
- nullable có thể biều diễn trong Union types như sau
public function foo(Foo|null $foo): void;
public function bar(?Bar $bar): void;
2. The nullsafe operator
- Before Php 8
Để hiện thì date ta phải thực hiện check nếu không muốn bắn ra exeption
$startDate = $dateAsString = $booking->getStartDate();
$dateAsString = $startDate ? $startDate->asDateTimeString() : null;
Hoặc một số framework như Laravel có hỗ trợ phương thức optional để thực hiện nullsafe operator
- Php 8:
$dateAsString = $booking->getStartDate()?->asDateTimeString();
3.Match expression
- Php 8 bạn có thể dùng match thay thế cho lệnh switch
switch ($statusCode) {
case 200:
case 300:
$message = null;
break;
case 400:
$message = 'not found';
break;
case 500:
$message = 'server error';
break;
default:
$message = 'unknown status code';
break;
}
$message = match ($statusCode) {
200, 300 => null,
400 => 'not found',
500 => 'server error',
default => 'unknown status code',
};
4. Named arguments
- Cho phép truyền data vào function mà không cần tuân theo thứ tự
function foo(string $a, string $b, ?string $c = null, ?string $d = null)
{ /* … */ }
foo(
b: 'value b',
a: 'value a',
d: 'value d',
);
5. Constructor property promotion
Thay vì phải khai báo contructor and property như sau
class Money
{
public Currency $currency;
public int $amount;
public function __construct(
Currency $currency,
int $amount,
) {
$this->currency = $currency;
$this->amount = $amount;
}
}
Php 8 làm nó gọn gàng và dễ nhìn hơn
class Money
{
public function __construct(
public Currency $currency,
public int $amount,
) {}
}
6. New static return type
class Foo
{
public function test(): static
{
return new static();
}
}
7. New mixed type
- mixed type bao gồm các kiểu sau:
array
bool
callable
int
float
null
object
resource
string
8. Throw expression
- Throw từ là 1 statement thành 1 expression
$triggerError = fn () => throw new MyError();
$foo = $bar['offset'] ?? throw new OffsetDoesNotExist('offset');
9. Inheritance with private methods
10. Allowing ::class on objects
- cho phép gọi ::class thay vì get_class()
$foo = new Foo();
var_dump($foo::class);
11. Non-capturing catches
- Trước Php 8 khi tất cả exception được lưu vào 1 biến vào bạn phải khải báo biến để chứa exception mặc dù nhiều lúc không dùng đến
try {
} catch (MySpecialException $exception) {
Log::error("Something went wrong");
}
Với Php 8 có thể làm viết như sau nếu không cần dùng $exception
try {
} catch (MySpecialException) {
Log::error("Something went wrong");
}
12. Trailing comma in parameter lists
- bạn có thể để thừa dấu phẩy ở cuối list param
public function(
string $parameterA,
int $parameterB,
Foo $objectfoo,
) {
// …
}
13. Tạo DateTime objects từ interface
DateTime::createFromInterface(DateTimeInterface $other);
DateTimeImmutable::createFromInterface(DateTimeInterface $other);
14. New str_contains() function
- Dùng str_contains(return bool) thay cho strpos(return int) để kiểm tra một chuột chưa một chuỗi khác
if (strpos('string with lots of words', 'words') !== false) { /* … */ }
if (str_contains('string with lots of words', 'words')) { /* … */ }
15. New str_starts_with() and str_ends_with() functions
str_starts_with('haystack', 'hay'); // true
str_ends_with('haystack', 'stack'); // true
16. Thay đổi Abstract methods khi sử dụng traits
trait Test {
abstract public function test(int $input): int;
}
Trước Php 8:
class UsesTrait
{
use Test;
public function test($input)
{
return $input;
}
}
Php 8:
class UsesTrait
{
use Test;
public function test(int $input): int
{
return $input;
}
}
17. Concatenation precedence
echo "sum: " . $a + $b;
- Trước Php 8: biểu thức trên sẽ được hiểu là:
echo ("sum: " . $a) + $b;
- Php 8
echo "sum: " . ($a + $b);
18. Attributes
- Cho phép thêm meta data cho class mà không cần sử dụng docblocks
use App\Attributes\ExampleAttribute;
@@ExampleAttribute
class Foo
{
@@ExampleAttribute
public const FOO = 'foo';
@@ExampleAttribute
public $x;
@@ExampleAttribute
public function foo(@@ExampleAttribute $bar) { }
}
@@Attribute
class ExampleAttribute
{
public $value;
public function __construct($value)
{
$this->value = $value;
}
}
Tham khảo : https://stitcher.io/blog/new-in-php-8