Đăng nhập bằng tài khoản google với laravel
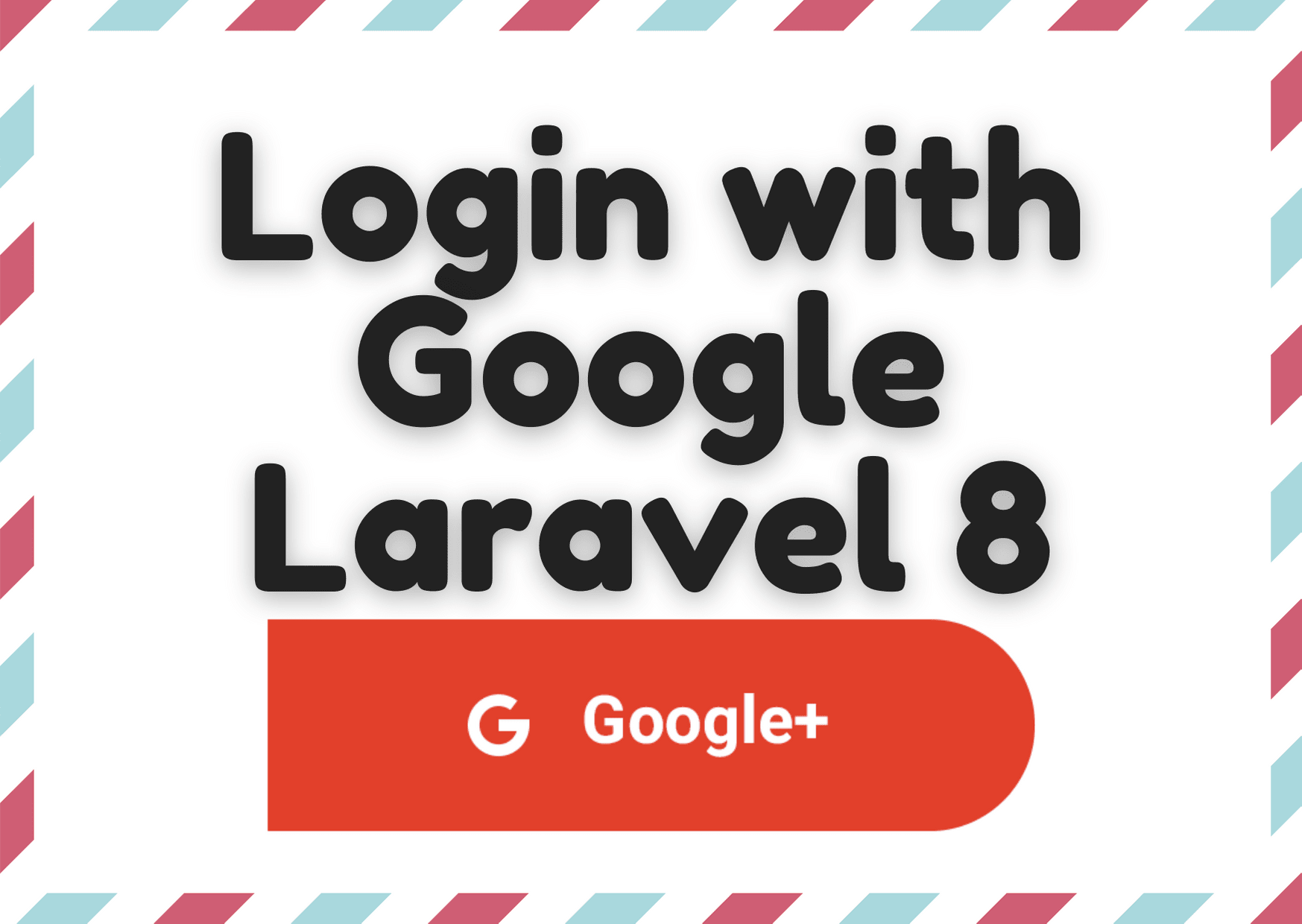
Giới thiệu
Xin chào các bạn, Trong ví dụ này, tôi sẽ hướng dẫn bạn cách đăng nhập bằng tài khoản google trên trang web laravel của bạn. trong ví dụ này, tôi sẽ sử dụng Socialite composer package để đăng nhập bằng tài khoản google gmail.
1.Cài đặt project
$ composer create-project laravel/laravel googlelogin
2.Setup cơ sở dữ liệu
- File .env trong project thiết lập các thông tin để kết nối đến csdl của bạn
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=databasename
DB_USERNAME=username
DB_PASSWORD=password
3.Cài đặt Socialite Package
Bây giờ cài đặt laravel Socialite Package để đăng nhập google. Mở cửa sổ command line với link ở project laravel ta vừa tạo ở mục 1 và chạy lệnh dưới đây:
composer require laravel/socialite
Sau khi cài đặt thành công gói socialite, ở trong config/app.php hãy thêm vào aliases và providers như sau:
'providers' => [
// Other service providers…
Laravel\Socialite\SocialiteServiceProvider::class,
],
'aliases' => [
// Other aliases…
'Socialite' => Laravel\Socialite\Facades\Socialite::class,
],
4.Cấu hình thông tin đăng nhập Google trong Laravel
- Thêm credentials vào config/services.php
'google' => [
'client_id' => env('GOOGLE_CLIENT_ID'),
'client_secret' => env('GOOGLE_CLIENT_SECRET'),
'redirect' => env('GOOGLE_REDIRECT')
],
5.Tạo ứng dụng của bạn trên google
- Tạo project: https://console.developers.google.com/projectcreate
- Tạo credentials: https://console.developers.google.com/apis/credentials
- Link tham khảo: https://www.youtube.com/watch?v=T8Jq3XE2sxo&ab_channel=ExpertRohila
khi tạo và cấu hình credentials xong thì google sẽ cung cấp cho chúng ta có dạng sau:
- Client ID = xxx9634542726-qlm1568arun8besekss2pitnkt8unpul.apps.googleusercontent.com
- Client secret = xxxmvJra33blkviub1_LIVA
- Url call back bạn config khi ở bước tạo credentials có dạng: http://localhost:8000/api/callback (ở local)
- Sau khi có được các thông số trên ta tiến hành cấu hình thêm ở file .env như sau:
GOOGLE_CLIENT_ID="xxx9634542726-qlm1568arun8besekss2pitnkt8unpul.apps.googleusercontent.com"
GOOGLE_CLIENT_SECRET="xxxmvJra33blkviub1_LIVA"
GOOGLE_REDIRECT="http://localhost:8000/api/callback"
6: Tạo migration
- Ở migration tạo bảng users ta thêm 1 trường google_id
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateUsersTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('google_id')->nullable();
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('users');
}
}
- Update model User:
app/Models/User.php
<?php
namespace App\Models;
use Illuminate\Contracts\Auth\MustVerifyEmail;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
class User extends Authenticatable
{
use HasFactory, Notifiable;
/**
* The attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'name',
'email',
'password',
'google_id',
];
/**
* The attributes that should be hidden for arrays.
*
* @var array
*/
protected $hidden = [
'password',
'remember_token',
];
/**
* The attributes that should be cast to native types.
*
* @var array
*/
protected $casts = [
'email_verified_at' => 'datetime',
];
}
7: Tạo route
- Do mình viết api lên mình tạo route trong: routes/api.php
<?php
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| API Routes
|--------------------------------------------------------------------------
|
| Here is where you can register API routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| is assigned the "api" middleware group. Enjoy building your API!
|
*/
Route::middleware('auth:api')->get('/user', function (Request $request) {
return $request->user();
});
// Google Sign In
Route::post('/get-google-sign-in-url', [\App\Http\Controllers\Api\GoogleController::class, 'getGoogleSignInUrl']);
Route::get('/callback', [\App\Http\Controllers\Api\GoogleController::class, 'loginCallback']);
8: Tạo controller
- chạy command line:
php artisan make:controller Api/GoogleController
- ở hàm loginCallback mình chỉ lấy thông tin lưu vào db, các bạn muốn redirect pass qua auth thì các bạn viết thêm xử lý ở trong hàm này của mình.
<?php
namespace App\Http\Controllers\Api;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Http\Response;
use App\Models\User;
use Laravel\Socialite\Facades\Socialite;
class GoogleController extends Controller
{
public function getGoogleSignInUrl()
{
try {
$url = Socialite::driver('google')->stateless()
->redirect()->getTargetUrl();
return response()->json([
'url' => $url,
])->setStatusCode(Response::HTTP_OK);
} catch (\Exception $exception) {
return $exception;
}
}
public function loginCallback(Request $request)
{
try {
$state = $request->input('state');
parse_str($state, $result);
$googleUser = Socialite::driver('google')->stateless()->user();
$user = User::where('email', $googleUser->email)->first();
if ($user) {
throw new \Exception(__('google sign in email existed'));
}
$user = User::create(
[
'email' => $googleUser->email,
'name' => $googleUser->name,
'google_id'=> $googleUser->id,
'password'=> '123',
]
);
return response()->json([
'status' => __('google sign in successful'),
'data' => $user,
], Response::HTTP_CREATED);
} catch (\Exception $exception) {
return response()->json([
'status' => __('google sign in failed'),
'error' => $exception,
'message' => $exception->getMessage()
], Response::HTTP_BAD_REQUEST);
}
}
}
9: Test login với postman
1: Gọi đến route với đường dẫn: "http://127.0.0.1:8000/api/get-google-sign-in-url" để lấy được url login.
2: Lấy kết quả url ở bước 1 paste sang 1 tab chrome khác để vào giao diện chọn account để đăng nhập của google
- Như vậy chúng ta đã hoàn thành việc đăng nhập bằng tài khoản google với laravel rồi.
Kết Luận
Ở bài này mình đã giới thiệu và hướng dẫn các bạn cách đăng nhập tài khoản google với laravel. Hy vọng bài viết đem lại cho các bạn nhiều điều hay ho. Có gì thắc mắc thì các bạn hãy comment ở dưới nhé . Và đừng quên upvote để mình có động lực với những bài tiếp theo nhé.